Quasar unit test with vitest - Quasar Mock
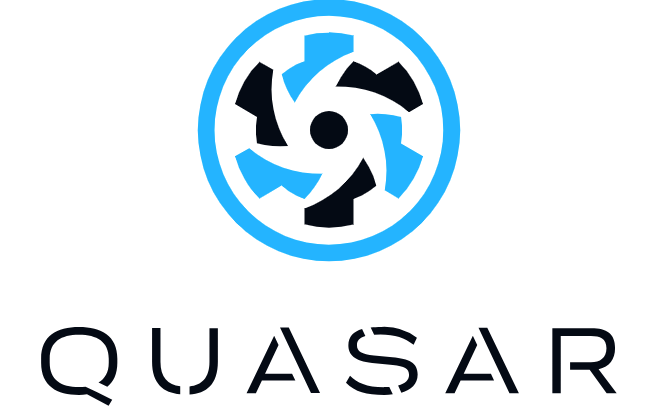
When we delve into unit tests, problems arise that perhaps few people have shared on the internet, in this case I am going to share a series of articles to be able to test multiple use cases, screen size, axios, vue router, vuex store, and more.
Quasar Mock
If you look up the noun mock in the dictionary, you will find that one of the definitions of the word is something made as an imitation.
Source: https://stackoverflow.com/a/2666006/8009061
First, I created a file called quasar.js
inside the /test/mocks
directory.
import { vi } from 'vitest'
/**
* Mocks Quasar, is a set of properties that are used in the components
* For example to set the screen size, the language, the theme, etc.
*/
export default {
screen: { gt: { xs: true } },
dark: { isActive: false },
iconSet: { arrow: { dropdown: 'arrow_drop_down' } },
lang: {
label: {
collapse: vi.fn(),
expand: vi.fn(),
"clear": "Clear",
"ok": "OK",
"cancel": "Cancel",
"close": "Close",
"set": vi.fn(),
"select": "Select",
"reset": "Reset",
"remove": "Remove",
"update": "Update",
"create": "Create",
"search": "Search",
"filter": "Filter",
"refresh": "Refresh"
}
},
notify: { mounted: vi.fn() },
}
Ideally, the mocks are in a separate directory, this gives organization and easy access. Here we see how to integrate our mock in a test.
After we have our Quasar mock, we can add properties like plugins, screen sizes, and whatever else we need into our tests.
import { describe, it, expect, vi } from 'vitest'
import { mount } from '@vue/test-utils'
import { Quasar } from 'quasar'
import $q from '../mocks/Quasar' // <-- The mock
import DesktopMenu from "../../src/components/DesktopMenu.vue";
const wrapperFactory = () => mount(DesktopMenu, {
global: {
plugins: [Quasar],
mocks: {
$q: {...$q} // Here the implementation of the mock
},
}
})
const wrapper = wrapperFactory();
describe('My component', () => {
it('mount component', () => {
expect(DesktopMenu).toBeTruthy();
})
})
Source: https://github.com/ta-vivo/ta-vivo/blob/master/tests/interface/DesktopMenu.test.js