Quasar unit test with vitest - i18n and the $t method
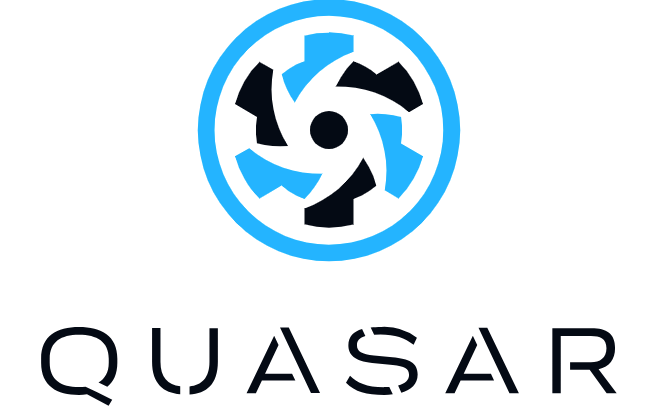
If you are using i18n
and tried to test a component where you use the $t
method, you have already encountered the _ctx.$t is not a function
error.
import { describe, it, expect } from 'vitest'
import { mount } from '@vue/test-utils'
import { Quasar } from 'quasar'
import Discord from "../../src/components/Integrations/Form/Discord.vue";
const wrapperFactory = () => mount(Discord, {
global: {
plugins: [Quasar]
}
})
const wrapper = wrapperFactory();
describe('Discord integration form', () => {
it('mount component', () => {
expect(Discord).toBeTruthy();
})
it('should render button to dispatch integration', () => {
const integrationButton = wrapper.find('button');
expect(integrationButton.exists()).toBe(true);
})
it('should contain example image', () => {
const exampleImage = wrapper.find('img');
expect(exampleImage.exists()).toBe(true);
})
})
This is solved in a very simple way, only need to add the $t
mock inside the component mount
import { describe, it, expect } from 'vitest'
import { mount } from '@vue/test-utils'
import { Quasar } from 'quasar'
import Discord from "../../src/components/Integrations/Form/Discord.vue";
const wrapperFactory = () => mount(Discord, {
global: {
plugins: [Quasar],
mocks: { // Here the solution
$t: (msg) => msg
}
}
})
const wrapper = wrapperFactory();
describe('Discord integration form', () => {
it('mount component', () => {
expect(Discord).toBeTruthy();
})
it('should render button to dispatch integration', () => {
const integrationButton = wrapper.find('button');
expect(integrationButton.exists()).toBe(true);
})
it('should contain example image', () => {
const exampleImage = wrapper.find('img');
expect(exampleImage.exists()).toBe(true);
})
})
With this simple code we can continue with our tests.